PDF generation is very important for business and in many business scenarios like invoice generation, agreement generation etc, we have to generate PDFs. We can generate PDFs using server-side (by Salesforce Apex) and client-side ( using lightning component and visual force pages). This post will explain how we can generate PDF using jSPDF in Lightning Web Component.
There are many client-side tools available for PDF generation using Javascript like jsPDF, PDFKit, pdfmake, Puppeteer, pdf-lib, pdfme. Due to lightning locker service, most client-side libraries are blocked. We can use jsPDF library but a few functionalities like HTML to pdf generation will not work in Salesforce as dependent libraries are blocked by lightning locker.
Let us see the steps to use jsPDF in Salesforce LWC.
- Add jsPDF libraries in Static Resources
- Use jsPDF in the Lightning Web component
- Test Component
1. Add jsPDF libraries in Static Resources
Download jsPDF (jspdf.umd.min.js) from its Github repository. After downloading add that file into Salesforce Static resource as jsPDF. Make sure it is added as public.
2. Use jsPDF in the Lightning Web component
once jsPDF is added to a static resource, we can include that library in Lightning Web Component using loadscript of platformResourceLoader.
Three steps are required to generate PDFs using jsPDF.
- Create jsPDF instance
- Add text, table, images, diagrams, etc.
- Save the file as PDF
a. Create jsPDF instance
To use jsPDF we have to create an instance of this library using jsPDF class. It supports default constructors as well as parameter constructors.
//Default constructor
var doc = new jsPDF();
//Paramter constructor for portrait pdf
const doc = new jsPDF('p', 'in', 'letter');
//using constructor with attribute
const doc =new jsPDF({ orientation: 'landscape', unit: 'in', format: [4, 2] });
b. Add text, table, images, diagrams, etc.
We can add text, tables, images, diagrams, and other elements to PDF documents.
//add text to PDF
doc.text(20, 20, 'Hello SalesforceCodex!');
doc.text(20, 30, 'jsPDF is client-side Javascript to generate a PDF.');
// Add new page
doc.addPage();
doc.text(20, 20, 'Visit SalesforceCodex.com');
//Sets the text color setTextColor(ch1, ch2, ch3, ch4)
doc.setTextColor(100);
There are a lot of other methods available for PDF generation. You can check those in the it’s documentation.
c. Save the file as a PDF
Once you put all code for document generation, you can call the save method to generate and save PDF files in the local system.
//Save File
doc.save("a4.pdf");
Complete Source Code
3. Test Component
Add the above-created component on the lightning page. It will show generate button as we are creating a PDF completely from Js code. We can use text from template control like a text box or rich text but we have to handle styles in the JS code itself. For this post I have used hardcoded text to put in PDF.
Here are two PDFs created using the above code.
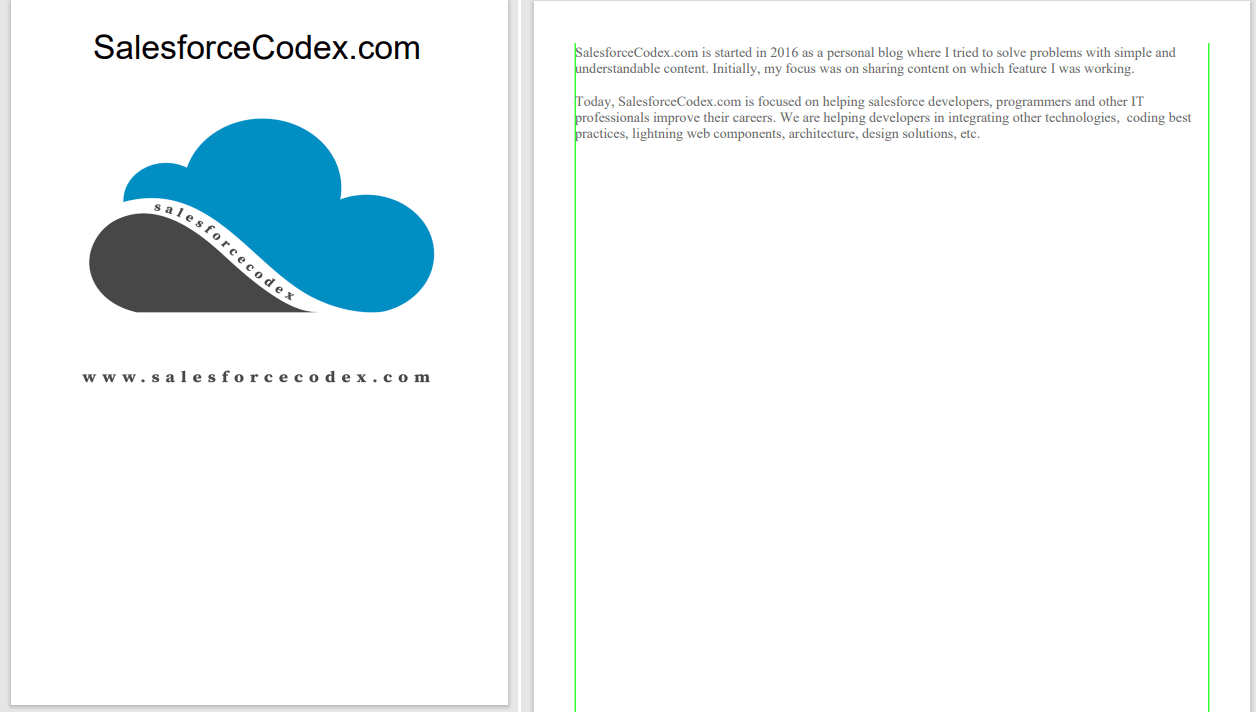
8 comments
Hi, I tried the code in my org but it is throwing the error as “Error TypeError: Cannot destructure property ‘jsPDF’ of ‘window.jspdf’ as it is undefined.”
Hello Surya,
Please check jsPdf is loading properly or not. As per the error, the library is not loaded correctly.
Thank You,
Dhanik
Hi,
Dhanik
How can i add an imagen using static source?
and also Can you please explicain how use table with fields related in pdf?
Thanks
Hello EdWard,
You can convert your images to base64 and add using addImage method. You can also check jsFiddle for image at https://jsfiddle.net/godcznLt/
You can check link for table example.
Thank You,
Dhanik
Hello im getting this Error: Lightning Web Security: platformResourceLoader error loading ‘/resource/1713097642000/jsPDF/jspdf.umd.min.js’.
Hello Hussain,
Please check that you have uploaded jsPDF file in static resource or not. If uploaded, then please check you are referring correct path like in your error it showing that you have created jsPDF folder and jspdf**.js file kept inside it. Is this correct?
Thank You,
Dhanik
Hello DHANIK
i get this Error: Lightning Web Security: platformResourceLoader error loading ‘/resource/1713097642000/jsPDF/jspdf.umd.min.js’.
(anonymous) @ aura_prod.js:102
Hello Rajish,
Please check that you have uploaded jsPDF file in static resource or not. If uploaded, then please check you are referring correct path like in your error it showing that you have created jsPDF folder and jspdf**.js file kept inside it. Is this correct?
Thank You,
Dhanik