When developing new features, some time we reach org character limit. In that case we have to remove unused code from Salesforce org. Let us create excel report of all custom metadata like custom field, apex classes, lightning component, flow etc from tooling api.
In our last post Find Referenced Metadata using Salesforce Dependency API dependency is checked for specific custom metadata.
This post will get list of all custom metadata in hierarchical grid. If metadata is used somewhere it will show detail where it is used.
Steps for getting complete metadata detail:
- Get list of All custom metadata using Tooling API
- Create Hierarchical data in Apex
- Use Lightning-tree-data for showing data
- Download complete data in CSV
1. Get list of All custom metadata using Tooling API
We can get complete list of custom metadata and it’s referenced object detail using MetadataComponentDependency Tooling API object. This object is still in beta version but it is available for Developer/Administrator after Summer 20 Release.
Select MetadataComponentId, MetadataComponentName, RefMetadataComponentName, RefMetadataComponentId, MetadataComponentType from MetadataComponentDependency
MetadataComponentId is id of metadata component which is dependent on another component RefMetadataComponentId.
IMPORTANT STEP:
We have to call Tooling API from Lightning Web Component so refer post CALL TOOLING API FROM LIGHTNING WEB COMPONENT. You can use Default scope refresh_token full in Auth Provider and Named Credential, if you don’t want to change user.
2. Create Hierarchical data in Apex
Based on above query we can get data from Tooling API. We can create hierarchical data in apex itself or in Lighting Web Component also. For this blog, I have created hierarchical data in apex.
We can use MetadataComponentId and RefMetadataComponentId for creating hierarchical data list.
//Parent Loop
for(MetadataRecord record:metadataRecords)
{
TreeNode node=new TreeNode();
node.MetadataComponentName=record.MetadataComponentName;
//Get Child or Reference
List<TreeNode> childs=new List<TreeNode>();
for(MetadataRecord rd:metadataRecords)
{
if(rd.MetadataComponentId==record.MetadataComponentId)
{
TreeNode nd=new TreeNode();
nd.MetadataComponentName=record.MetadataComponentName;
childs.add(nd);
}
}
node.children=childs;
}
As we have custom metadata list and we need to get child or dependent custom objects within same list. For this we can use loop to get dependent of each custom object.
3. Use Lightning-tree-data for showing data
Now we have hierarchical data in list from above step. Let us show this complete data in lightning-tree-grid object. This component displays structured data in a table with expandable rows.
<lightning-tree-grid
columns={columns}
data={gridData}
key-field="MetadataComponentName"
></lightning-tree-grid>
Add column for lightning tree grid.
export const COLUMNS_DEFINITION = [
{
type: 'text',
fieldName: 'MetadataComponentName',
label: 'Metadata Name',
initialWidth: 300,
},
......
@track columns=COLUMNS_DEFINITION;
lightning-tree-grid need child record as _children object and in apex we can not create property starting with underscore(_) so we have to transform children as _children object for each metadata record.
var result=[];
data.forEach(child => {
var ch=JSON.parse(JSON.stringify(child));
if(ch.children!==undefined && ch.children.length>0)
{
ch._children=ch.children;
}
ch.children=null;
result.push(ch);
});
this.gridData=result;
4. Download complete data in CSV
We can download all metadata record which is shown in lightning-tree-grid. We can create string data variable and based on required column we can add comma(,) in string record variable.
this.gridData.forEach(function(rowArray) {
let row = rowArray.MetadataComponentName+","+rowArray.RefMetadataComponentType+","+rowArray.RefMetadataComponentName+",";
if(rowArray._children!==undefined)
{
rowArray._children.forEach(function(child) {
csvContent += row + "\r\n";
row = child.MetadataComponentName+","+child.RefMetadataComponentType+","+child.RefMetadataComponentName+",";
});
}
csvContent += row + "\r\n";
});
Complete code:
Apex:
Lightning Web Component
Test Page
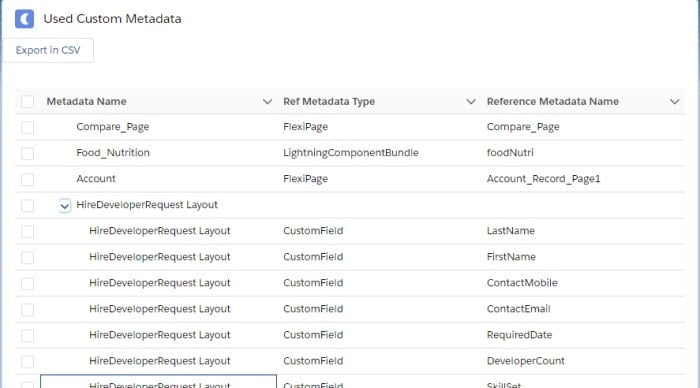
References:
https://developer.salesforce.com/docs/component-library/bundle/lightning-tree-grid/example
https://releasenotes.docs.salesforce.com/en-us/summer20/release-notes/rn_feature_impact.htm
Related Posts
Salesforce DevOps for Developers: Enhancing Code Quality and Deployment Efficiency
Apex Code Coverage In Custom Object
Get All Used Custom Metadata Detail
Find Referenced Metadata using Salesforce Dependency API
Extract list of all fields from Page Layout